A pointer is a variable which stores memory addresses. Because there are no pass-by reference, we use pointers to emulate pass by reference: by sharing addresses with other functions.
A pointer can identify a single byte OR some large data structures. We can dynamically allocate pointers, and also identify memory generically without types.
C is always pass-by-copy. Therefore, to pass-by-reference, you basically have to
int x = 2; // declare object
int *xptr = &x; // get location of object (&: address of)
printf("%d\n", *xptr); // dereference the pointer
address operator
You will note, in the line above:
int *xptr = &x;
uses an operator &
to get the address of an object. That’s called an object operator.
pointer memory diagram
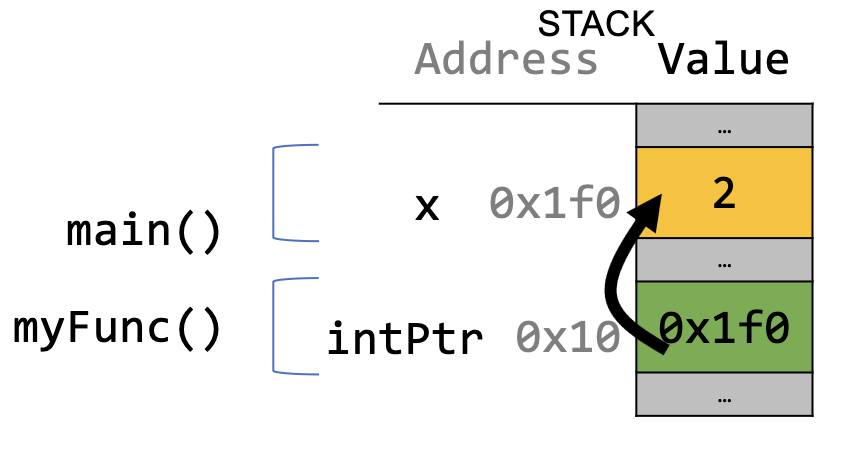
void myFunct(int *intPtr) {
*intPtr = 3;
}
int main() {
int x = 2;
myFunct(&x);
}