In C, string is an array of chars. C strings don’t track their length; each C string always end in an null-terminating character: \0
. This is represents the zero byte.
There’s a built in function strlen
which checks the length of a string without the null-terminating character. This function is O(n)
!!!
String Pointer Syntax Sugar Synonyms
char str[6];
// these are equivalent
char *ptr = str;
char *ptr = &str[0];
char *ptr = &str; // DON'T DO THIS
// these are equivalent
char thirdLetter = str[3];
char thirdLetter = *(str + 3);
seven commandments of c strings
- if we create a string as
char[]
, we can modify its characters because its memory lives in our stack instead of living in a global data segment - we can’t set
char[]
as equaling to something, because its not strictly a pointer and instead it refers to an entire block of memory instead of a pointer to the first element (in a same vein, an array’s size is fixed and travels with the variable) - if we pass
char[]
as a parameter, it is converted to achar *
- if we create a string with new string literal as
char *thing = "thing"
, we can’t modify it because its on the global data segment - we can set
char *
equaling to another value because its a pointer - adding an offset to a c string gives a substring that’s places past the first character
- if we change characters in a string parameter, these changes will persist
passing strings around
Strings are passed as a pointer to their first character.
void foo(char *str) {
// do string things
}
char string[6]; // THIS IS A STRING OF LENGTH 5!!!! (beacuse there's a null terminator)
foo(string); // pass the syntax sugar pointer
foo(&string[0]); // pass the actual first pointer
you won’t know whether or not this is the address to a string or a pointer to a single character; so good practice to call it something_str
if you’d like a string.
character manipulation checker
#include <ctype.h>
int main() {
isalpha(ch);
islower(ch);
...
}
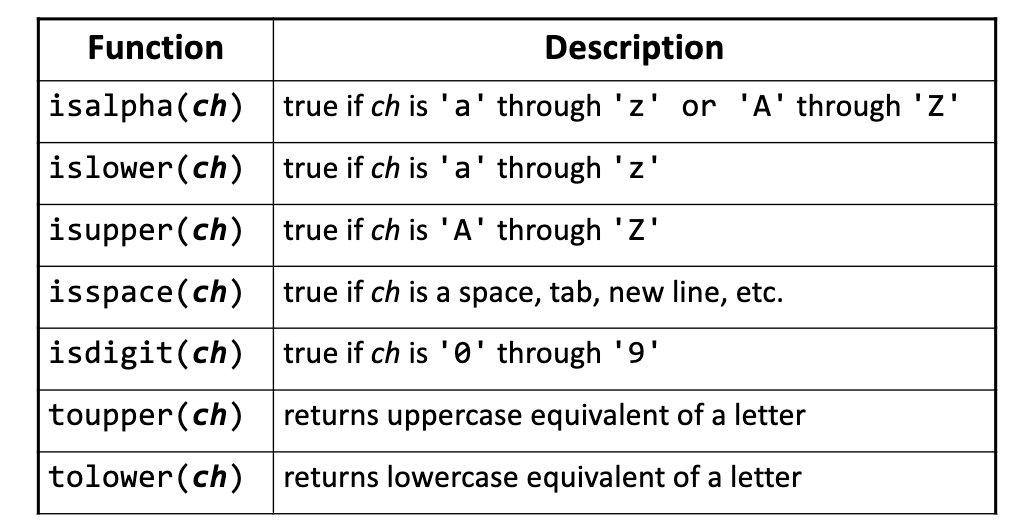
string manipulations
#include <string.h>
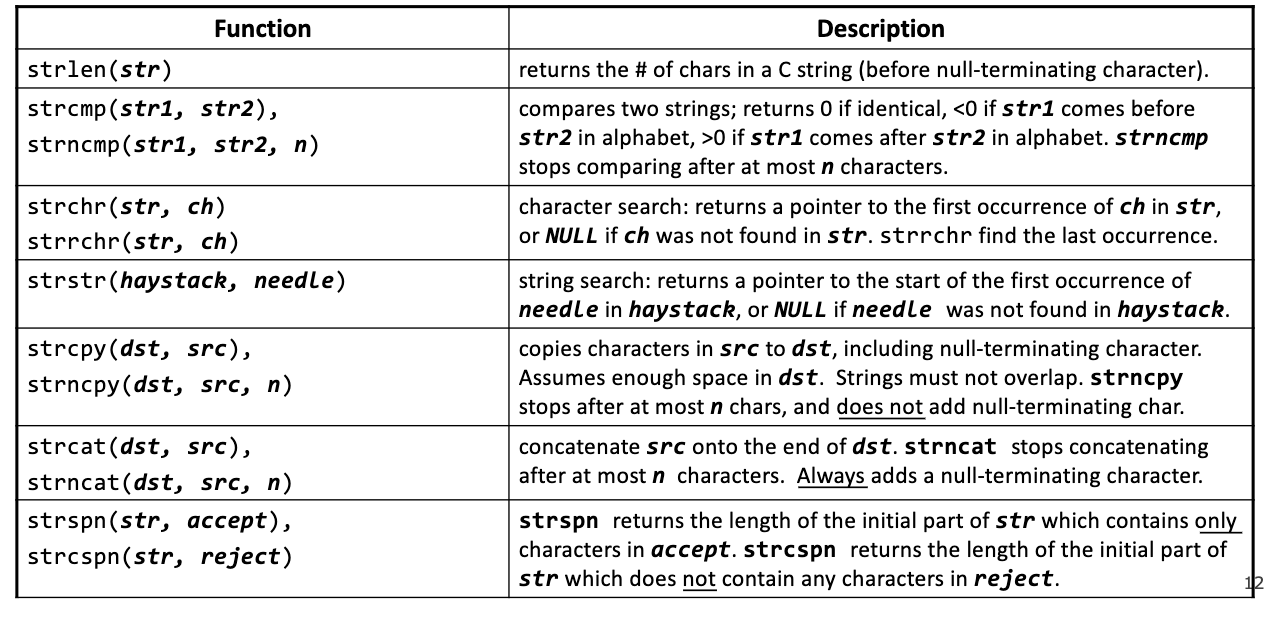
strcmp
When you comparing strings, you can’t use == or < or >. Instead:
#include <string.h>
int main() {
int cmp = strcmp(str1, str2);
if (cmp == 0) {
// if str1 is equal to str2
} else if (cmp < 0) {
// if str1 comes before str2 lexographically
} else {
// if str2 comes before str1 lexographically
}
}
strcpy
Copying strings, dangerously, because buffer overflows are fun.
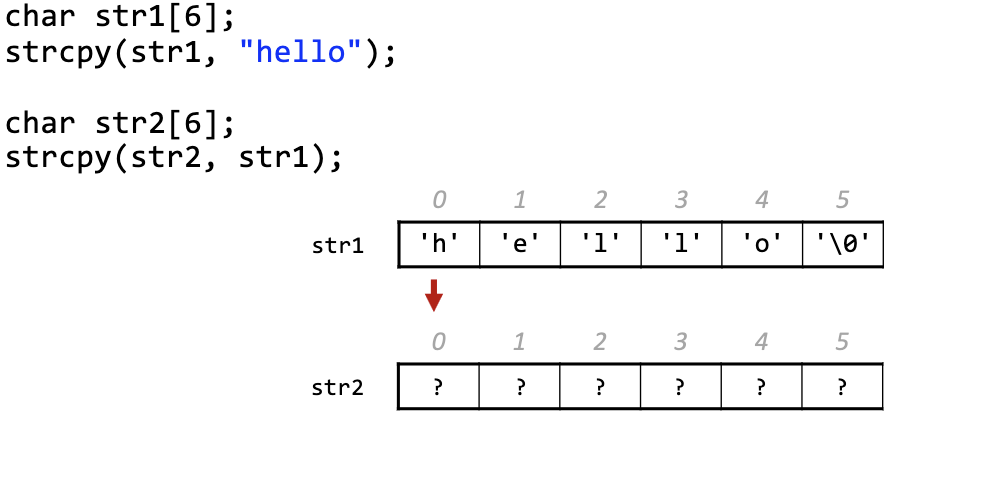
This function does NOT care about buffer overflows, and WILL put in a null terminator.
strncopy
This function optimize against buffer overflow, but it may not write a null terminator.
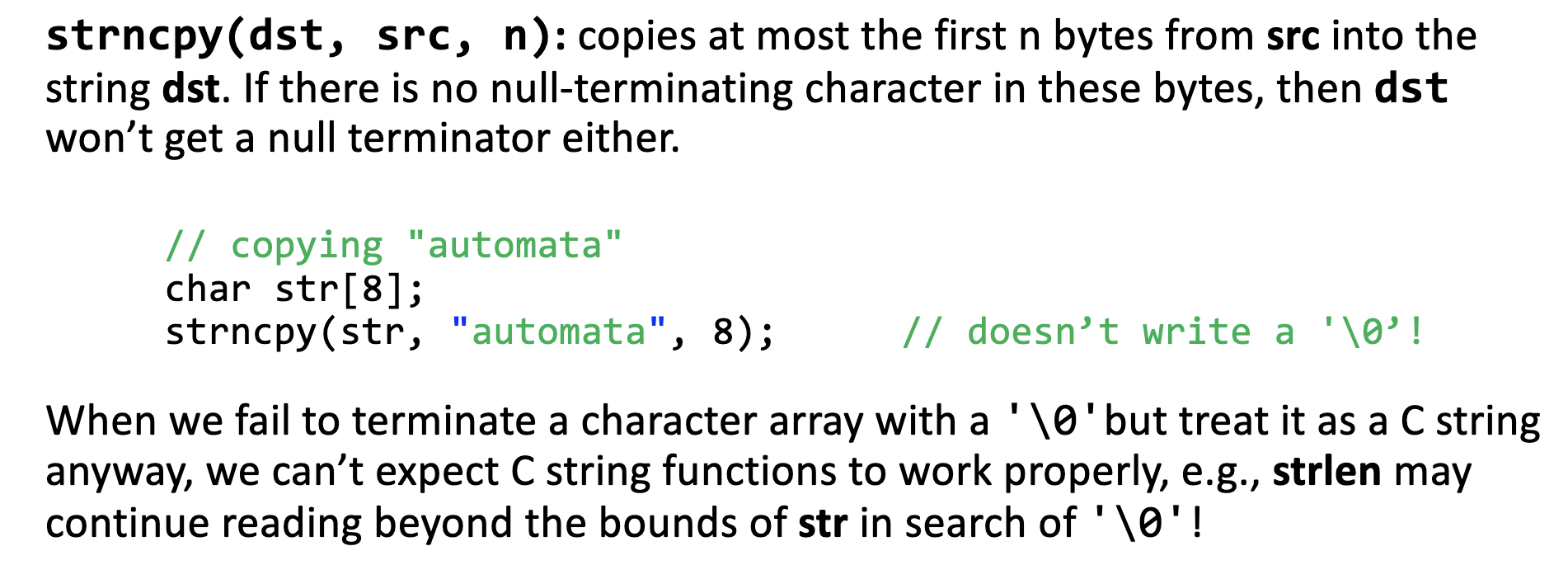
strcat
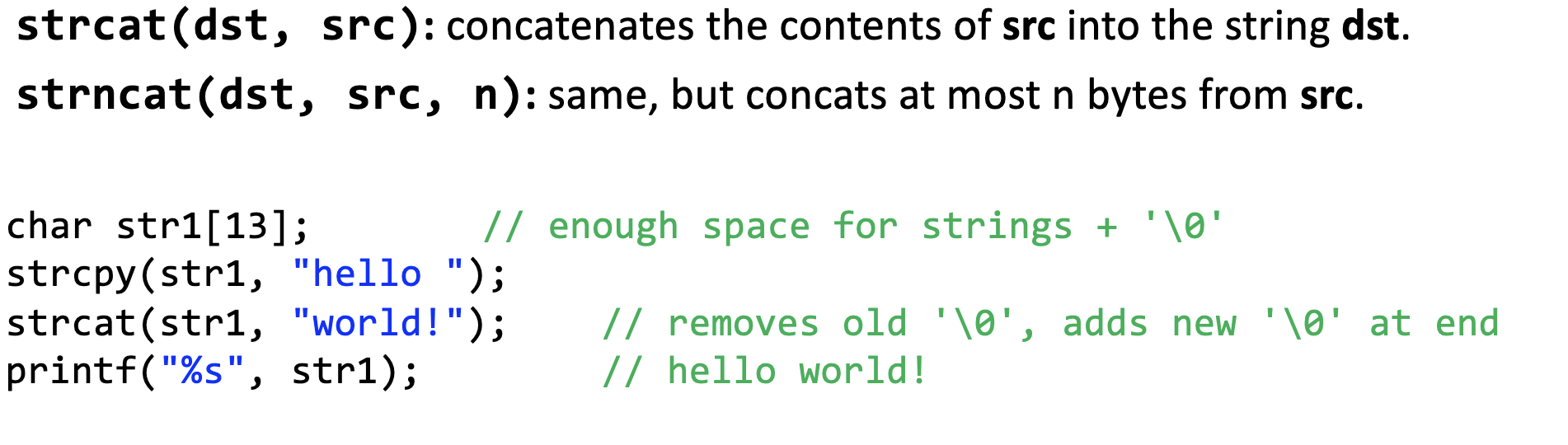
strncat always puts in a null terminator.
pointer arithmetic with strings
Fortunatly, each char is
strspn
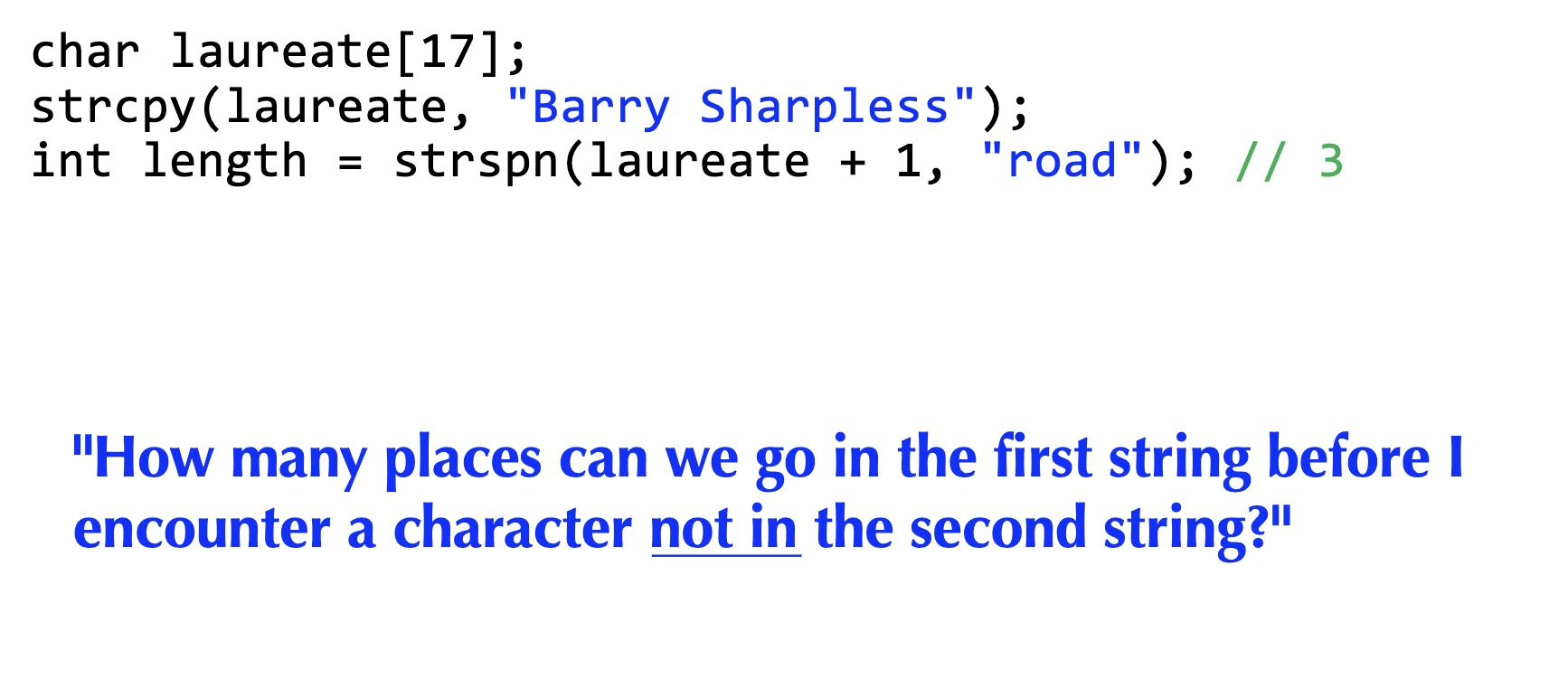
Count the number of characters that are “cool”: contained within the end